Parameterizing the class in c++
Several languages the notion of a parameterizing the class in c++, or template is noticable.
This concept is most obviously useful for working with groups in a strongly typed language. By this way, in general we can define behavior for sets by defining a template class Set.
class Set <T> { void insert (T newElement); void remove (T anElement);
When we have done this, we can use the general definition to make set classes for more specific elements.
Set <Employee> employeeSet;
We declare a parameterized class in the UML using the notation shown in Figure 6-18.

The T in the diagram is a placeholder for the type parameter. (You may have more than one.) In an untyped language, such as Smalltalk, this issue does not come up, so this concept is not useful.
A use of a parameterized class, such as Set<Employee>, is called a bound element.
We can show a bound element in two ways. The first way mirrors the C++ syntax (see below figure)
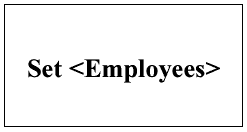
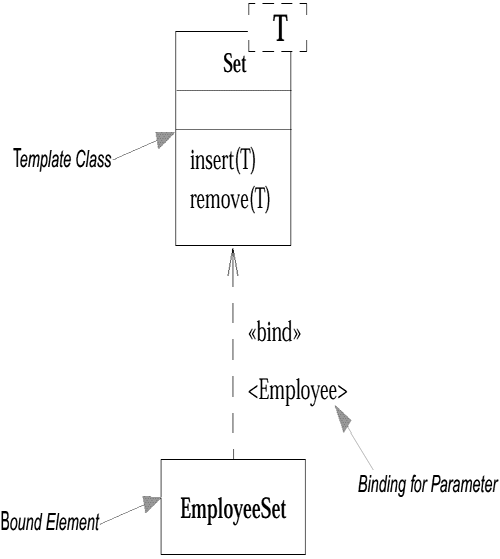
Using a bound element is not the same as subtyping. However, We are not allowed to add features to the bound element, which is completely specified by its template; we are adding only restricting type information. If we want to add features, we must create a subtype.
Parameterized classes allow us to use a derived typing. When we write the body of the template, we may invoke operations on the parameter. When we later declare a bound element, the compiler tries to ensure that the supplied parameter supports the operations required by the template.
This is a derived typing mechanism because we do not have to define a type for the parameter; the compiler figures out whether the binding is viable, by looking at the source of the template. This property is central to the use of parameterized classes in C++’s standard template library (STL); Someother interesting tricks are also used in these classes.