continue statement in c
The continue statement in C programming language works somewhat like the breakstatement. Instead of forcing termination, however, continue forces the next iteration of the loop to take place, skipping any code in between.
For the for loop, continue statement causes the conditional test and increment portions of the loop to execute. For the while and do…while loops, continue statement causes the program control passes to the conditional tests.
Syntax:
The syntax for a continue statement in C is as follows:
continue;
Flow Diagram:
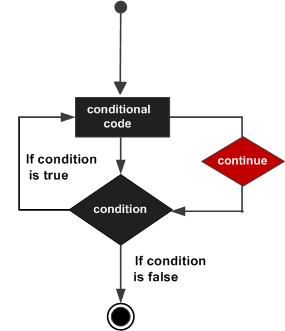
continue statement
Example:
#include <stdio.h> int main () { /* local variable definition */ int a = 10; /* do loop execution */ do { if( a == 15) { /* skip the iteration */ a = a + 1; continue; } printf("value of a: %d\n", a); a++; }while( a < 20 ); return 0; }
When the above code is compiled and executed, it produces the following result:
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 16 value of a: 17 value of a: 18 value of a: 19
for more click here…..